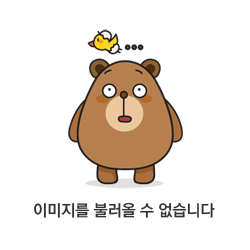
개요
플러터 어플리케이션을 작성하다 보면, 상황에 맞게 발생하는 에러를 적절히 구분하여 처리할 필요성을 강하게 느낍니다. 이 글에서는 try- catch 구문과 관련 코드를 예로 들어 에러를 적절히 처리하는 방법을 서술합니다.
비동기 함수 실행 시간 제한
간혹, 사용하는 비동기 함수가 내부 설계 문제로 에러를 반환하지 않고 계속 요청을 보내는 경우가 있습니다. 이 경우를 대비하기 위해 해당 비동기 함수 실행 시간을 어느 정도 제한할 필요가 있습니다.
아래 예제는 unIntendedFuntion 의 요청 후 3초가 지나도 응답이 없는 경우, TimeoutException 에러를 반환하도록 설계하였습니다.
test () async {
try {
await unIntendedFunction().timeout(
const Duration(seconds: 3),
onTimeout: () => throw const TimeoutException('서버 응답 시간이 초과되었습니다.'),
);
} catch (Error e) {
}
}
이 때, Catch 블록에서 TimeoutException 에러를 감지하여 에러 반환 시 전달한 message (서버 응답 시간이 초과되었습니다.) 를 출력할 수 있습니다.
test () async {
try {
await unIntendedFunction().timeout(
const Duration(seconds: 3),
onTimeout: () => throw const TimeoutException('서버 응답 시간이 초과되었습니다.'),
);
} catch (Error e) {
if(e == TimeoutException) {
print('Error message : ${e.message}');
}
}
}
사용 가능한 에러 종류
위 예제에선 TimeoutException 에러 객체를 반환했습니다. 실제로 플러터에서 지원, 사용가능한 에러 종류는 더 많습니다. 자세한 목록은 플러터 공식 홈페이지를 참고하세요.
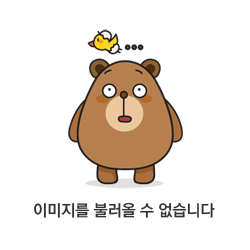
직접 에러를 정의하여 사용할 수도 있습니다. 아래는 geolocator 플러그인 내부에서 정의된 LocationServiceDisabledException 에러 정의 코드입니다.
/// An exception thrown when trying to access the device's location
/// information while the location service on the device is disabled.
class LocationServiceDisabledException implements Exception {
/// Constructs the [LocationServiceDisabledException]
const LocationServiceDisabledException();
@override
String toString() => 'The location service on the device is disabled.';
}
아래와 같이 기존과 동일한 방식으로 LocationServiceDisabledException 에러를 반환할 수 있습니다.
permission = await Geolocator.checkPermission();
if (permission == LocationPermission.denied) {
permission = await Geolocator.requestPermission();
if (permission == LocationPermission.denied) {
throw const LocationServiceDisabledException();
}
}
참고 자료
'프로그래밍 > Flutter <Dart>' 카테고리의 다른 글
[Flutter] Firebase Analytics 연동하기 (0) | 2025.02.13 |
---|---|
[Flutter] iOS Invalid Pre-Release Train. The train version '1.0' is closed for new build submissions 문제 해결하기 (0) | 2025.01.12 |
[Flutter] 안드로이드 배포 시 프로젝트 버전 수정하기 (0) | 2025.01.12 |
[Flutter/android] 플러터에 안드로이드 앱 링크 적용하기 with Go Router (0) | 2024.12.07 |
[Flutter] 플러터 안드로이드 apk 설치 오류 해결하기 (0) | 2024.12.04 |